In modern software development, achieving efficient and consistent test automation requires leveraging advanced technologies and practices. Integrating Docker with Jenkins for executing Playwright tests provides a powerful combination that ensures reproducible test environments, scalability, and streamlined CI/CD processes.
This detailed tutorial will guide you through setting up Jenkins for running Playwright tests inside Docker containers, with Java as the example language.
We will also explore advanced scenarios and optimizations to make your testing environment robust and efficient.
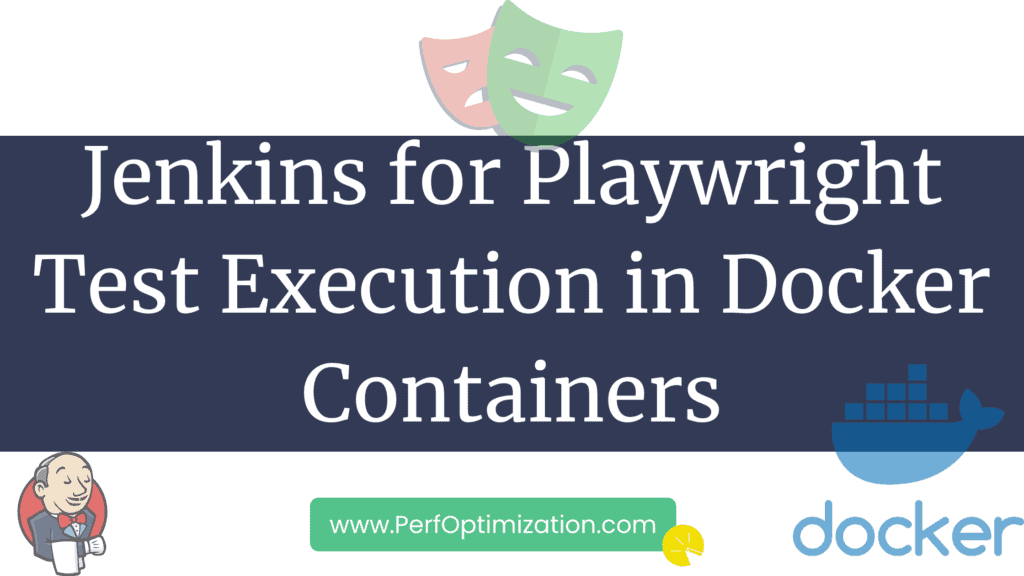
- 1. Prerequisites
- 2. Setting Up Docker for Playwright
- 3. Creating a Playwright Test Suite in Java
- 4. Configuring Jenkins for Docker Integration
- 5. Setting Up a Jenkins Pipeline for Playwright Tests
- 6. Optimizing Docker and Jenkins for Test Execution
- 7. Advanced Docker and Jenkins Configurations
- 8. Conclusion
1. Prerequisites
Before setting up Jenkins with Docker and Playwright, ensure you have the following:
- Jenkins: A Jenkins instance installed and running, accessible via a web interface.
- Docker: Docker installed and configured on the Jenkins server, with appropriate permissions.
- Java Development Kit (JDK): Version 11 or later installed on your development machine and Docker container.
- Playwright for Java: Installed in your project, compatible with your Java version.
- Maven: Installed as a build tool (though Gradle can be used similarly).
- Basic Knowledge: Familiarity with Docker, Jenkins, and Playwright.
2. Setting Up Docker for Playwright
To execute Playwright tests within Docker containers, you need to prepare a Docker image that includes Java, Playwright, and all necessary dependencies.
A. Creating a Dockerfile
A Dockerfile
defines the environment and instructions for building a Docker image. Create a Dockerfile
in your project’s root directory:
# Use an official OpenJDK runtime as a parent image
FROM openjdk:11-jre-slim
# Install required system dependencies
RUN apt-get update && apt-get install -y \
curl \
unzip \
wget \
git \
libx11-dev \
libxcomposite1 \
libxdamage1 \
libxrandr2 \
libxss1 \
libxtst6 \
libnss3 \
libatk-bridge2.0-0 \
libgtk-3-0 \
libgbm1 \
&& apt-get clean \
&& rm -rf /var/lib/apt/lists/*
# Install Node.js and npm
RUN curl -fsSL https://deb.nodesource.com/setup_18.x | bash - \
&& apt-get install -y nodejs
# Install Playwright
RUN npm install -g playwright
# Install Playwright browsers
RUN playwright install
# Set the working directory
WORKDIR /app
# Copy Maven build files
COPY pom.xml /app/
# Install Maven dependencies
RUN mvn dependency:go-offline -B
# Copy the rest of the application
COPY src /app/src/
# Build the Maven project
RUN mvn clean install
# Set the entry point to run Playwright tests
ENTRYPOINT ["mvn", "test"]
This Dockerfile starts from a base image with Java, installs necessary system libraries, and sets up Playwright. It also includes steps to build your Maven project and install Playwright browsers.
B. Building the Docker Image
Build the Docker image using the Dockerfile:
docker build -t playwright-java-image .
This command creates a Docker image named playwright-java-image
with all dependencies and Playwright installed.
3. Creating a Playwright Test Suite in Java
To test Playwright within Docker containers, create a Java test suite. Start by adding Playwright dependencies to your pom.xml
:
<dependency>
<groupId>com.microsoft.playwright</groupId>
<artifactId>playwright</artifactId>
<version>1.35.0</version>
</dependency>
Then, create a Playwright test class:
import com.microsoft.playwright.*;
public class PlaywrightTest {
public static void main(String[] args) {
try (Playwright playwright = Playwright.create()) {
Browser browser = playwright.chromium().launch(new BrowserType.LaunchOptions().setHeadless(true));
Page page = browser.newPage();
page.navigate("https://example.com");
System.out.println("Title: " + page.title());
page.screenshot(new Page.ScreenshotOptions().setPath(Paths.get("screenshot.png")));
}
}
}
Place this test in the src/test/java
directory. It launches a Chromium browser, navigates to a URL, prints the page title, and takes a screenshot.
4. Configuring Jenkins for Docker Integration
To execute Docker containers from Jenkins, configure Jenkins to use Docker and set up a pipeline for running Playwright tests.
A. Installing Docker Plugin for Jenkins
- Go to Manage Jenkins > Manage Plugins.
- Search for Docker and install the Docker Commons Plugin.
B. Configuring Docker in Jenkins
- Go to Manage Jenkins > Configure System.
- Scroll down to the Docker section.
- Add a new Docker cloud configuration and provide your Docker server details.
C. Setting Up Docker as a Build Agent
- Go to Manage Jenkins > Manage Nodes and Clouds.
- Click on New Node.
- Create a Permanent Agent and configure it to use Docker as its build environment.
5. Setting Up a Jenkins Pipeline for Playwright Tests
To automate Playwright test execution, set up a Jenkins Pipeline. Create a Jenkinsfile
in the root of your project repository:
pipeline {
agent {
docker {
image 'playwright-java-image'
label 'docker'
}
}
stages {
stage('Checkout') {
steps {
git 'https://github.com/your-repo-url.git'
}
}
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('Run Playwright Tests') {
steps {
sh 'mvn test'
}
}
stage('Publish Reports') {
steps {
junit '**/target/surefire-reports/*.xml'
allure includeProperties: false, results: [[path: 'target/allure-results']]
}
}
}
post {
always {
cleanWs()
}
}
}
Explanation
- agent: Defines the Docker image to use for running the pipeline.
- stages: Contains steps to check out code, build the project, run tests, and publish test reports.
- post: Cleans up the workspace after each build.
C. Configuring Jenkins Pipeline
- Create a new Pipeline job in Jenkins.
- Point it to your
Jenkinsfile
in the Git repository. - Trigger the job to ensure the pipeline executes successfully.
6. Optimizing Docker and Jenkins for Test Execution
Optimizing Docker and Jenkins can enhance the performance and reliability of your test execution.
A. Caching Dependencies
To reduce build time, cache dependencies between builds. Modify your Dockerfile to cache Maven dependencies:
# Cache Maven dependencies
COPY pom.xml /app/
RUN mvn dependency:go-offline -B
This step ensures that Maven dependencies are only downloaded if pom.xml
changes.
B. Parallel Test Execution
Playwright supports parallel test execution to speed up testing. Configure parallel tests in your Playwright setup:
import com.microsoft.playwright.*;
public class ParallelPlaywrightTest {
public static void main(String[] args) {
try (Playwright playwright = Playwright.create()) {
Browser browser = playwright.chromium().launch(new BrowserType.LaunchOptions().setHeadless(true));
// Run multiple tests in parallel
Page page1 = browser.newPage();
Page page2 = browser.newPage();
page1.navigate("https://example.com");
page2.navigate("https://example.org");
// Additional test logic
}
}
}
C. Resource Management
Ensure Docker containers have sufficient resources. Configure Docker’s resource limits to allocate enough CPU and memory:
docker run --memory="2g" --cpus="2.0" -t playwright-java-image
This command sets memory to 2GB and allocates 2 CPUs for the container.
7. Advanced Docker and Jenkins Configurations
A. Customizing Docker Networking
For more control over how Docker containers interact, customize Docker networking:
- 1. Create a Docker Network:
docker network create my-network
2. Use the Network in Docker Compose or Docker Run:
docker run --network my-network -t playwright-java-image
This setup allows containers to communicate within the same network.
B. Scaling Test Execution
Use Kubernetes to scale test execution. Kubernetes can dynamically scale the number of test containers based on workload.
- Set Up Kubernetes Plugin for Jenkins: Install the Kubernetes plugin in Jenkins to integrate with your Kubernetes cluster.
- Configure Kubernetes for Jenkins: Define Jenkins agents as Kubernetes Pods and configure them to run Playwright tests. This setup enables you to scale your test infrastructure based on demand.
C. Using Jenkins Pipelines for Test Reporting
Enhance test reporting by aggregating results and visualizing them in Jenkins:
- Aggregate Results: Modify your
Jenkinsfile
to aggregate test results:
stage('Aggregate Results') {
steps {
sh 'cp -r test-results/* combined-results/'
}
}
Visualize Results:
Use Jenkins plugins such as Test Results Analyzer for improved visualization and insights into test results.
8. Conclusion
Integrating Playwright with Docker and Jenkins provides a powerful solution for automated testing, offering scalability, consistency, and efficiency. By following this guide, you’ve set up a robust testing framework that includes advanced configurations like parallel test execution, resource management, and Kubernetes scaling.