Writing robust automation scripts involves not just creating functional tests but also anticipating and handling errors effectively.
For any automation framework where interactions with browsers and web pages are key, error handling and debugging techniques can significantly enhance script reliability and maintainability. In this article, we’ll learn more about how Error Handling and Debugging in Playwright Scripts works.
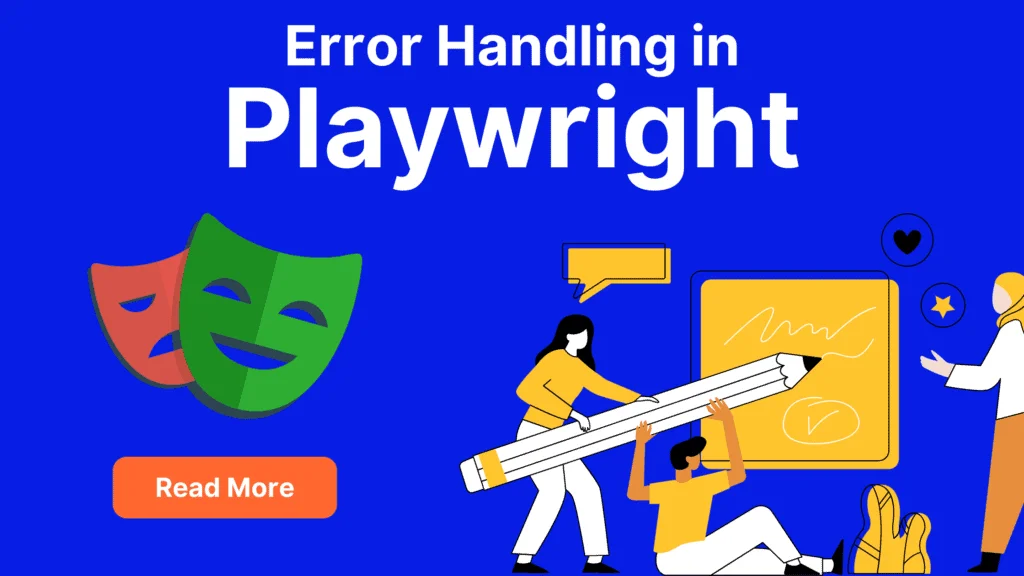
Common Errors Encountered in Playwright
1. Selector Errors:
Playwright relies on selectors to interact with elements on web pages. Errors commonly occur when:
- Incorrect Selector: Using an incorrect or invalid CSS selector or XPath expression.
- Element Not Found: Attempting to interact with an element that doesn’t exist or hasn’t loaded yet.
- Dynamic Content: Selecting elements that dynamically load after a delay or an action.
try {
await page.click('nonexistentSelector');
} catch (error) {
console.error('Error clicking element:', error);
}
2. Network Errors:
Interaction with network requests often leads to errors due to:
- Request Failures: Failed requests due to timeouts, network issues, or unavailability of resources.
- Intercepted Requests: Errors can occur if the handling logic isn’t robust when intercepting requests.
page.on('requestfailed', (request) => {
console.error(`Request ${request.url()} failed`);
});
3. Page Errors:
Errors can originate from the loaded web page itself, including:
- JavaScript Errors: Occurrence of JavaScript errors on the page due to code issues or conflicts.
- Unhandled Promises: Unhandled promises or asynchronous operations causing failures.
page.on('pageerror', (error) => {
console.error('Page error occurred:', error);
});
4. Navigation Errors:
Errors can arise during page navigation or browser interactions such as:
- Navigation Failures: Failing to navigate to a specific URL or encountering navigation timeouts.
- Browser Context Issues: Issues related to browser context creation or switching.
try {
await page.goto('invalidURL');
} catch (error) {
console.error('Error navigating:', error);
}
5. Timeout Errors:
Timeouts are common in Playwright due to:
- Page Load Timeout: Failure to load a page within a specified timeout period.
- Action Execution Timeout: Timing out while waiting for an action (click, type) to complete.
try {
await page.waitForSelector('element', { timeout: 5000 });
} catch (error) {
console.error('Timeout waiting for element:', error);
}
Effective Error Handling Techniques
1. Try-Catch Blocks:
Encapsulating critical Playwright interactions within try-catch blocks helps in catching and handling errors gracefully. This approach ensures that a script doesn’t abruptly halt due to unforeseen errors.
Nested Try-Catch: For more complex scenarios or multiple interactions, nesting try-catch blocks can isolate errors at different stages of script execution.
try {
await page.click('button');
} catch (error) {
console.error('Error clicking button:', error);
}
2. Logging Errors:
Utilize logging mechanisms to capture errors along with contextual information, aiding in better understanding and debugging. Logging can include:
- Console Output: Leveraging
console.error()
orconsole.log()
to output error details and intermediate checkpoints during script execution. - Custom Logging: Implementing a custom logging mechanism or integrating with logging frameworks to store error details, timestamps, and relevant script context.
try {
// Playwright interactions
} catch (error) {
console.error('Error occurred:', error);
// Additional logging or handling logic
}
3. Retry Mechanisms:
Implementing retry logic for specific operations helps in addressing transient errors or intermittent failures. This technique is especially useful for dealing with flaky elements or network issues.
Exponential Backoff: Consider implementing an exponential backoff strategy, increasing the delay between retry attempts to reduce load on the application under test.
async function clickElementWithRetry(selector, retries = 3) {
for (let i = 0; i < retries; i++) {
try {
await page.click(selector);
return; // Exit function on successful click
} catch (error) {
console.error('Error clicking element:', error);
}
}
console.error(`Failed to click element ${retries} times`);
}
4. Custom Error Handling Functions:
Creating custom error-handling functions or modules allows for centralized error processing and standardized error reporting across scripts. This helps in maintaining consistency and ease of modification.
Error Recovery Strategies: Develop strategies to recover from specific errors dynamically within these custom error handlers, enabling script continuation or alternate actions.
function handlePageError(error) {
console.error('Page error occurred:', error);
// Additional error handling or reporting logic
}
page.on('pageerror', handlePageError);
Debugging Playwright Scripts
Debugging in Playwright is crucial for identifying, isolating, and resolving issues that might arise during script development or execution. Here are several techniques and tools to effectively debug Playwright scripts:
1. Logging and Output:
Strategic Console Output: Place console.log()
statements strategically at different stages of script execution to log intermediate values, variables, or checkpoints.
console.log('Starting script execution...');
// Playwright interactions
console.log('Completed script execution.');
2. Headful Mode and Slow Motion:
- Headful Mode: Run Playwright in non-headless mode (
{ headless: false }
) to visually observe script execution in a visible browser window, facilitating real-time observation of interactions. - Slow Motion: Introduce a delay (
{ slowMo: 50 }
) between actions to slow down script execution, making it easier to follow the sequence of interactions.
const browser = await chromium.launch({ headless: false, slowMo: 50 });
3. Using DevTools:
Interactive Browser Debugging: Integrate Playwright with DevTools to debug browser instances interactively, allowing inspection, breakpoints, and real-time interaction with the loaded page.
// Open DevTools on page load
page.on('DOMContentLoaded', () => {
page.keyboard.down('Control');
page.keyboard.press('F12');
page.keyboard.up('Control');
});
4. Debugging in IDEs:
Integrated Development Environments (IDEs): Leverage the debugging capabilities of IDEs (like VSCode, WebStorm) by setting breakpoints, stepping through code, and inspecting variables during script execution.
5. Error Stack Traces and Logging:
- Error Stack Traces: Analyze detailed error stack traces provided by the Playwright to pinpoint the exact line or operation that caused the error, aiding in rapid issue identification.
- Custom Logging for Errors: Implement specialized error logging for capturing and storing error details, including timestamps and contextual information for easier diagnosis.
6. Conditional Breakpoints and Assertions:
- Conditional Breakpoints: Set breakpoints in the script with conditional statements to halt execution at specific points when certain conditions are met.
- Assertions: Include assertions within the script to validate expected outcomes, helping to detect discrepancies or unexpected behavior during execution.
7. Remote Debugging and Tracing:
- Remote Debugging: Utilize Playwright’s remote debugging capabilities to debug scripts running on remote machines or devices, enabling comprehensive troubleshooting.
- Tracing and Profiling: Employ tracing and profiling tools to analyze performance bottlenecks, resource utilization, and execution timelines for script optimization.
Conclusion:
Error handling and debugging are integral parts of creating stable and resilient Playwright scripts. By proactively addressing potential errors and employing effective debugging strategies, developers can streamline the development process and ensure the reliability of their automated tests.
Remember, understanding the context of errors and utilizing appropriate debugging techniques can significantly enhance the quality and efficiency of your Playwright scripts.
Happy scripting and debugging!
https://playwright.dev/docs/writing-tests